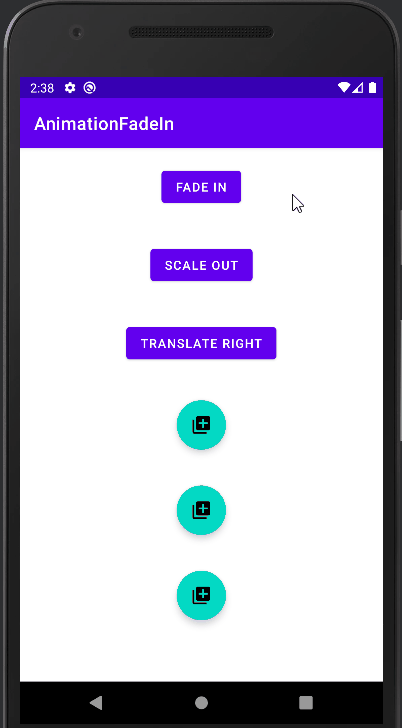
[Android/Kotlin] Animation Programmatically - alpha, translate, scale
Notepad96
·2022. 8. 25. 23:26
1. 요약
이번 글에서는 Animation을 코드로(programmatically) 혹은 Animation Resource File로 정의한 후 적용하는 방법에 관하여 기술한다.
구현한 Animation으로는 다음과 같이 3가지가 있다.
- alpha : 투명도를 조절하며, 투명한 상태에서 점점 불투명하게 함으로써 점점 나타나게 할 수 있음
- scale : 크기를 변화시키며, 크기가 0인 상태에서 점점 커지게 할 수 있음
- translate : 대상을 이동시키며, 시작과 종료 위치에 따라서 방향을 조절할 수 있음
2. 레이아웃
2-1. activity_main.xml
메인 레이아웃으로서 일반 Button 3개와, Floating Button 3개를 배치하였다.
일반 Button 3개에서는 코드로 정의한 Animation을 적용하고, Floating Button 3개에는 정의한 Animation Resource 파일의 Animation을 적용할 것이다.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center_horizontal"
tools:context=".MainActivity">
<Button
android:id="@+id/button01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginVertical="20dp"
android:text="Fade In" />
<Button
android:id="@+id/button02"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginVertical="20dp"
android:text="Scale Out" />
<Button
android:id="@+id/button03"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginVertical="20dp"
android:text="Translate Right" />
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/floatBtn01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginVertical="20dp"
android:src="@drawable/ic_baseline_add_to_photos_24"
/>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/floatBtn02"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginVertical="20dp"
android:src="@drawable/ic_baseline_add_to_photos_24"
/>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/floatBtn03"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginVertical="20dp"
android:src="@drawable/ic_baseline_add_to_photos_24"
/>
</LinearLayout>
3. 코드 및 설명
3-1. MainActivity.kt
각 Animation을 구현하기 위하여 AlphaAnimation, ScaleAnimation, TraslateAnimation 각 인스턴스를 정의하였다.
각 파라미터에 대응하는 값은 각 파라미터의 이름을 보면 알 수 있다.
(파라미터 정보를 보기 위해서는 괄호 안에서 "Ctrl + P"를 눌러준다. )
duration은 지속 시간으로 해당 애니메이션을 동작할 때 지속시간을 나타낸다.
package com.notepad96.animationfadein
import android.os.Bundle
import android.view.View
import android.view.animation.AlphaAnimation
import android.view.animation.AnimationUtils
import android.view.animation.ScaleAnimation
import android.view.animation.TranslateAnimation
import androidx.appcompat.app.AppCompatActivity
import com.notepad96.animationfadein.databinding.ActivityMainBinding
class MainActivity : AppCompatActivity() {
private val binding: ActivityMainBinding by lazy { ActivityMainBinding.inflate(layoutInflater) }
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(binding.root)
val fadeIn01 = AlphaAnimation(0.0f, 1.0f).apply {
duration = 3000
}
val scaleOut01 = ScaleAnimation(0f, 2f, 0f, 2f, .5f, .5f).apply {
duration = 2000
}
val moveRight01 = TranslateAnimation(0f, 300f, 0f, 0f).apply {
duration = 2000
}
binding.button01.setOnClickListener {
binding.button01.startAnimation(fadeIn01)
}
binding.button02.setOnClickListener {
binding.button02.startAnimation(scaleOut01)
}
binding.button03.setOnClickListener {
binding.button03.startAnimation(moveRight01)
}
val fadeIn02 = AnimationUtils.loadAnimation(applicationContext, R.anim.fade_in)
val scaleOut02 = AnimationUtils.loadAnimation(applicationContext, R.anim.scale_out)
val moveRight02 = AnimationUtils.loadAnimation(applicationContext, R.anim.move_right)
binding.floatBtn01.setOnClickListener {
binding.floatBtn01.startAnimation(fadeIn02)
}
binding.floatBtn02.setOnClickListener {
binding.floatBtn02.startAnimation(scaleOut02)
}
binding.floatBtn03.setOnClickListener {
binding.floatBtn03.startAnimation(moveRight02)
}
}
}
Animation을 정의하는 파일로서 drawable/anim 디렉터리의 파일을 생성한다. anim 디렉터리가 없을 경우 Android Resource Directory를 통하여 생성해 주면 된다.
이렇게 정의한 파일은 AnimationUtils.loadAnimation을 사용하여 초기화 후 사용 가능하다.
● alpha (anim/fade_in.xml)
투명도를 조절하는 기능으로서 투명한 상태에서 점점 나타나도록 Animation을 구현할 수 있다.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/decelerate_interpolator">
<alpha
android:fromAlpha="0.0"
android:toAlpha="1.0"
android:duration="1000"/>
</set>
● scale (anim/scale_out.xml)
크기를 조절하는 Animation으로서 fromScale = 0에서 toScale = 2로 지정하여 점점 커지도록 구현하였다.
pivot X, Y를 50%, 50%로 줌으로써 가운데에서 점점 나타나도록 하였으며, duration을 2000으로 줌으로써 2초 동안 해당 애니메이션이 동작하도록 한다.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<scale
android:pivotX="50%"
android:pivotY="50%"
android:fromXScale="0"
android:fromYScale="0"
android:toXScale="2"
android:toYScale="2"
android:duration="2000" />
</set>
● translate (anim/move_right.xml)
이동시키는 Animation을 보여줄 수 있으며 fromX = -200%에서 toX = 0%로 함으로써 왼쪽에서 오른쪽으로 이동하여 나타나듯이 구현하였다.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<translate android:fromXDelta="-200%"
android:toXDelta="0%"
android:duration="1000"/>
</set>
4. 전체 파일
GitHub - Notepad96/BlogExample02
Contribute to Notepad96/BlogExample02 development by creating an account on GitHub.
github.com
'Android' 카테고리의 다른 글
[Android/Kotlin] RecyclerView Divider - 구분선 만들기 (0) | 2022.08.27 |
---|---|
[Android/Kotlin] RecyclerView Move to Top - 최상단 이동 (2) | 2022.08.26 |
[Android/Kotlin] RecyclerView Last Item Check - 리스트 마지막 항목 (0) | 2022.08.24 |
[Android/Kotlin] DataBinding 데이터 바인딩 (0) | 2022.08.21 |
[Android/Kotlin] TabLayout Custom Style - 탭 스타일 (0) | 2022.08.20 |