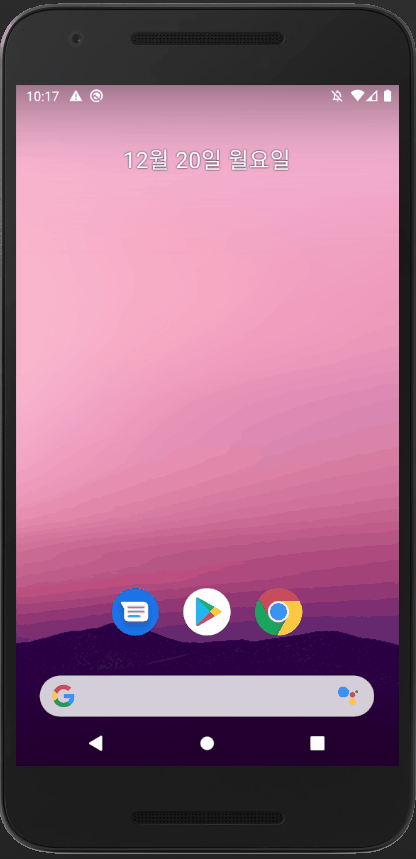
Android Kotlin Splash Page - 시작 페이지, 로고 페이지
Notepad96
·2021. 12. 20. 23:57
1. 결 과
# 해당 글은 Splash 화면을 구성하는 방법을 설명한다.
# Splash 화면은 애플리케이션을 실행하였을 때 수초간 회사의 CI를 보여주거나 애플리케이션을 대표하는 아이콘/이미지를 보여주는 화면을 지칭한다.
2. activity_splash.xml (Splash 화면 레이아웃)
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".SplashActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="252dp"
android:background="@drawable/ic_launcher_background"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/ic_launcher_foreground" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="4dp"
android:text="Splash Test"
android:textSize="22sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
</androidx.constraintlayout.widget.ConstraintLayout>
# 예시 Splash 화면으로 ImageView 1개와 TextView 1개로 구성하였다.
3. AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.splashpage">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.SplashPage">
<activity
android:name=".MainActivity"
android:exported="false" />
<activity
android:name=".SplashActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
# 애플리케이션의 처음으로 보여줄 시작 페이지는 splash 엑티비티의 레이아웃이여야함으로 Menifest파일에서 intent-filter로 시작 엑티비티로 지정해 준다.
4. SplashActivity.kt
package com.example.splashpage
import android.content.Intent
import android.os.Bundle
import android.os.Handler
import androidx.appcompat.app.AppCompatActivity
class SplashActivity : AppCompatActivity() {
private val DELAY_TIME = 1500L
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_splash)
Handler().postDelayed( {
startActivity(Intent(this, MainActivity::class.java))
finish()
} , DELAY_TIME)
}
}
# postDelayed를 사용함으로써 DELAY_TIME 만큼 경과 했을 때 startActivity와 finish를 실행한다.
# 엑티비티가 전환되기 전 splash 엑티비티의 화면이 DELAY_TIME만큼 보여진 후 엑티비티가 전환되므로 잠깐 보여줄 화면을 splash activity의 구성하면 된다.
※ DELAY_TIME은 ms로 1500은 1.5초간 화면을 보여준다.
5. 전체 코드
GitHub - Notepad96/BlogExample
Contribute to Notepad96/BlogExample development by creating an account on GitHub.
github.com
'Android' 카테고리의 다른 글
Android Kotlin Context Menu - 컨텍스트 메뉴 (0) | 2021.12.22 |
---|---|
Android Kotlin Option Menu - 옵션 메뉴 (0) | 2021.12.21 |
Android Kotlin SharedPreferences - 데이터 저장 (0) | 2021.12.19 |
Android Kotlin programmatically button style - 동적 버튼 스타일 적용 (0) | 2021.12.08 |
Android Kotlin Button Style - outline, text button (0) | 2021.12.07 |