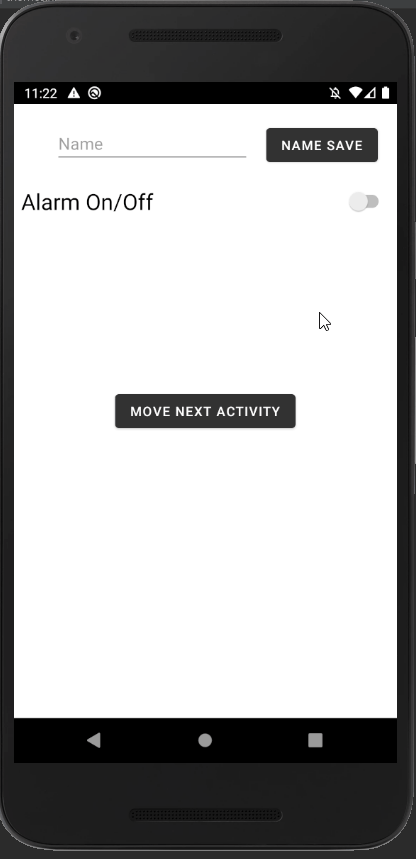
Android Kotlin SharedPreferences - 데이터 저장
Notepad96
·2021. 12. 19. 13:05
1. 결 과
# 해당 글은 SharedPreferences을 사용하여 데이터를 저장하고 불러와 사용하는 내용을 담고 있다.
# SharedPreferences는 데이터를 저장할 수 있으며 여기서 이 데이터는 옵션 값과 같은 가벼운 데이터를 저장하는 데 사용하는 것이 권장된다.
# 동일한 형식이 반복되는 다수의 값을 저장하여 무거워질 경우에는 DB를 사용하여야 한다.
Android Kotlin DB - Room을 사용하여 데이터베이스 사용 (1)
1. Room Room은 SQLite에 대하여 추상화 레이어를 제공하여 원활한 데이터베이스 액세스를 지원하는 동시에 SQLite를 완벽하게 활용할 수 있게한다. 따라서 공식 문서에서도 SQLite 대신 Room을 사용할 것
notepad96.tistory.com
# SharedPreferences는 해당 애플리케이션 내부의 저장되므로 애플리케이션을 삭제하면 저장된 값도 같이 삭제되어 버린다.
2. Layout
2-1. activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/vBtnMoveActivity"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Move Next Activity"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Switch
android:id="@+id/vSwitchAlarm"
android:layout_width="0dp"
android:layout_height="65dp"
android:layout_marginTop="72dp"
android:text="Alarm On/Off"
android:textSize="24sp"
android:padding="8dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/vEditUserName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="44dp"
android:layout_marginTop="20dp"
android:ems="10"
android:hint="Name"
android:inputType="textPersonName"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/vBtnNameSave"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:text="Name Save"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.46"
app:layout_constraintStart_toEndOf="@+id/vEditUserName"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
activity_main.xml은
# 이름을 입력하는 EditText 1개와 이를 저장하는 데 사용하는 Button 1개
# 알람의 On/Off 여부를 지정하는 Switch 1개
# activity_main2로 전환하는 데 사용하는 Button 1개
로 구성된다.
2-2. activity_main2.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity2">
<TextView
android:id="@+id/vTextResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="TextView"
android:textSize="20sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
activity_main2.xml은
# 저장된 값을 불러와 보여줄 수 있는 TextView 1개
로 구성된다.
3. MainActivity.kt
package com.example.sharedpreferences
import android.content.Intent
import android.content.SharedPreferences
import android.os.Bundle
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
import androidx.core.content.edit
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
lateinit var option: SharedPreferences
lateinit var userInfo: SharedPreferences
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
option = getSharedPreferences("option", MODE_PRIVATE)
userInfo = getSharedPreferences("user_info", MODE_PRIVATE)
vBtnMoveActivity.setOnClickListener {
var intent = Intent(this, MainActivity2::class.java)
startActivity(intent)
}
vBtnNameSave.setOnClickListener {
userInfo.edit {
putString("name", vEditUserName.text.toString())
vEditUserName.setText("")
}
Toast.makeText(applicationContext, "Name Save!!", Toast.LENGTH_LONG).show()
}
vSwitchAlarm.setOnCheckedChangeListener { compoundButton, b ->
option.edit {
putBoolean("alarm", vSwitchAlarm.isChecked)
}
}
}
override fun onStart() {
saveValueLoad() // Value Load
super.onStart()
}
private fun saveValueLoad() {
vEditUserName.setText(userInfo.getString("name", ""))
vSwitchAlarm.isChecked = option.getBoolean("alarm", false)
}
}
# SharedPreferences은 getSharedPreferences을 사용하여 초기화할 수 있다.
# getSharedPreferences 1번째 파라미터로 name이 들어가며 이를 사용하여 저장될 값들을 분류할 수 있다.
ex) user_info로 1차적으로 분류되었으면 이곳에 user_name, user_age의 값들을 저장함으로써 관리를 할 수 있다.
# getSharedPreferences 2번째 파라미터로 mode가 들어가며 MODE_PRIVATE를 사용하면 해당 앱에서만 접근 가능하다. (지정한 모드의 따라서 해당 액티비티에서만 접근 가능할 수도 있음)
# SharedPreferences를 초기화하였다면 edit과 putString, putBoolean와 같은 메서드를 사용하여 Key-Value 형태로 값을 저장할 수 있다.
# 저장된 값을 불러올 때는 SharedPreferences의 getString, getBoolean와 같은 메소드를 사용하면 되며 1번째 파라미터는 key값 2번째 파라미터는 값을 불러오지 못할 경우의 defalut 값을 지정한다.
4. MainActivity2.kt
package com.example.sharedpreferences
import android.content.SharedPreferences
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main2.*
class MainActivity2 : AppCompatActivity() {
lateinit var option: SharedPreferences
lateinit var userInfo: SharedPreferences
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main2)
option = getSharedPreferences("option", MODE_PRIVATE)
userInfo = getSharedPreferences("user_info", MODE_PRIVATE)
var str = """User Name: ${userInfo.getString("name", "NULL")}
|
|Alarm On/Off: ${boolToText(option.getBoolean("alarm", false))}
""".trimMargin()
vTextResult.text = str
}
fun boolToText(check: Boolean): String {
return when(check) {
true -> "On"
else -> "Off"
}
}
}
# SharedPreferences을 마찬가지로 name을 지정하여 getSharedPreferences로 초기화 하여준다.
# String값은 getString 메서드를 Boolean값은 getBoolean 메서드를 사용하여 저장된 값을 읽어온다.
5. 전체 코드
GitHub - Notepad96/BlogExample
Contribute to Notepad96/BlogExample development by creating an account on GitHub.
github.com
'Android' 카테고리의 다른 글
Android Kotlin Option Menu - 옵션 메뉴 (0) | 2021.12.21 |
---|---|
Android Kotlin Splash Page - 시작 페이지, 로고 페이지 (0) | 2021.12.20 |
Android Kotlin programmatically button style - 동적 버튼 스타일 적용 (0) | 2021.12.08 |
Android Kotlin Button Style - outline, text button (0) | 2021.12.07 |
Android Kotlin Snackbar - 안내 메시지 표시 (0) | 2021.11.01 |