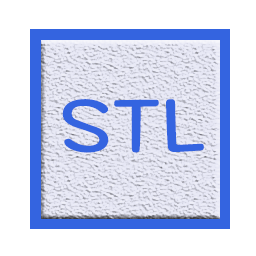
[STL] Multimap 생성, 삽입, 삭제 등 사용법
Notepad96
·2020. 11. 6. 23:00
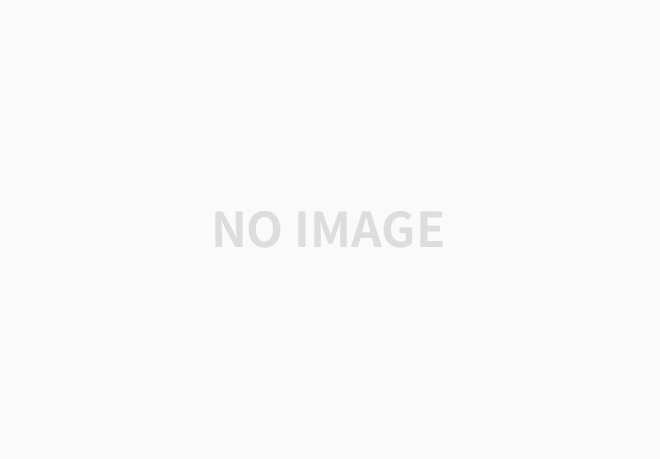
1. multimap
multimap 또한 set과 multiset과의 관계와 마찬가지로 map과는 중복되는 원소를 갖을 수 있다는 차이점만이 있다.
따라서 map에서 사용하였던 insert, erase, count, find와 같은 함수들을 그대로 사용할 수 있다.
단, map에서 insert 시 [] 연산자를 사용할 수 있었다면 multimap에서는 [] 연산자를 사용할 수 없다.
따라서 삽입 시 insert 멤버 함수를 사용하여서 pair 객체를 넣어주거나 해야 한다.
또한, find 멤버 함수는 똑같은 key값의 여러 원소가 존재하여도 맨 앞에 오는 원소의 반복자를 반환한다.
따라서 해당 key값의 모든 원소들의 접근하고자 한다면 multiset에서 사용하였던 것처럼 lower_bound()와 upper_bound() 멤버 함수를 사용한다면 할 수 있다.
[STL] Multiset 생성, 삽입, 삭제 등 사용법
1. multiset multiset은 중복된 원소를 저장할 수 있다는 점 외에는 set과 동일한 특성을 갖는다. multiset 또한 기본적으로 오름차순으로 정렬되며 조건자로 greater를 사용함으로써 내림차순으로 정렬
notepad96.tistory.com
2. 코 드
#include <iostream>
#include <map>
using namespace std;
int main() {
/* 생성자 */
//multimap<int, int> m; // <key, value> key 오름차순, 기본 값 : less<int>
multimap<int, int, greater<int>> m;
/* 삽입, 삭제 */
m.insert(pair<int, int>(2, 20)); // pair객체를 생성하여 삽입
m.insert(make_pair(3, 30));
m.insert(make_pair(1, 10));
m.insert(make_pair(2, 20));
m.insert(make_pair(3, 40));
m.insert(make_pair(5, 50));
m.insert(make_pair(4, 60));
m.erase(5);
/* 접근 */
for (pair<int, int> ii : m) {
cout << ii.first << ":" << ii.second << " ";
}
cout << "\n";
for (auto it = m.begin(); it != m.end(); it++) {
cout << it->first << ":" << it->second << " ";
}
cout << "\n";
cout << "key 3 개수 : " << m.count(3) << "\n";
/* 탐색 */
//auto it = m.find(5);
map<int, int>::iterator it = m.find(3); // key 3 원소 중 맨 앞의 반복자 갖는다.
if ( it == m.end()) {
cout << "해당 key는 존재하지 않습니다.\n";
}
else {
cout << "key " << it->first << "중 맨 앞 값은 : " << it->second << "\n";
}
// key = 3인 원소들 탐색
for (auto it = m.lower_bound(3); it != m.upper_bound(3); it++) {
cout << it->first << ":" << it->second << " ";
}
cout << "\n";
return 0;
}
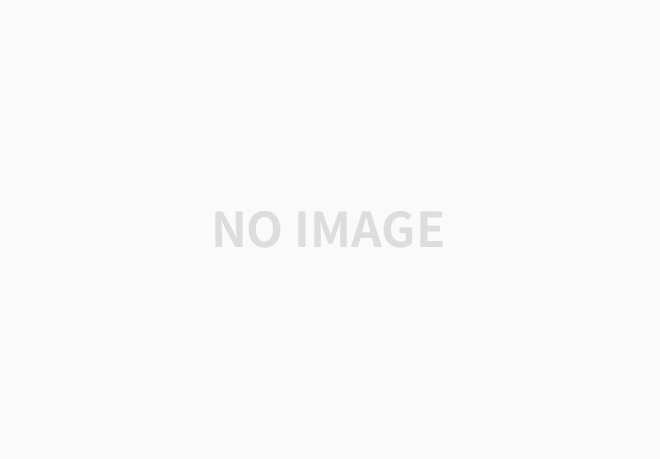
- multimap은 [] 연산자를 지원하지 않으므로 insert 함수를 사용하여 삽입하여야 한다.
- count 함수를 사용하여 해당 key의 해당하는 원소가 몇 개 존재하는지 개수를 구할 수 있다.
- find 함수의 결과 해당 key값의 해당하는 원소의 맨 앞을 가리키는 반복자를 리턴해준다.
따라서 해당 key의 해당하는 모든 원소를 구하고 싶을 경우 lower_bound(), upper_bound()를 사용하면 해당 원소들의 접근할 수 있다.
3. 참 조
multimap - C++ Reference
difference_typea signed integral type, identical to: iterator_traits ::difference_type usually the same as ptrdiff_t
www.cplusplus.com
'C++ > Container' 카테고리의 다른 글
[STL] Unordered_map, multimap 생성, 삽입, 삭제 등 사용법 (0) | 2020.11.08 |
---|---|
[STL] Unordered_set, multiset 생성, 삽입, 삭제 등 사용법 (1) | 2020.11.07 |
[STL] Map 생성, 삽입, 삭제 등 사용법 (0) | 2020.11.06 |
[STL] Multiset 생성, 삽입, 삭제 등 사용법 (0) | 2020.11.05 |
[STL] Set 생성, 삽입, 삭제 등 사용법 (0) | 2020.11.05 |