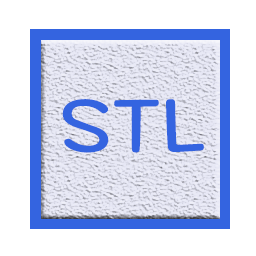
[STL] Multiset 생성, 삽입, 삭제 등 사용법
Notepad96
·2020. 11. 5. 20:51
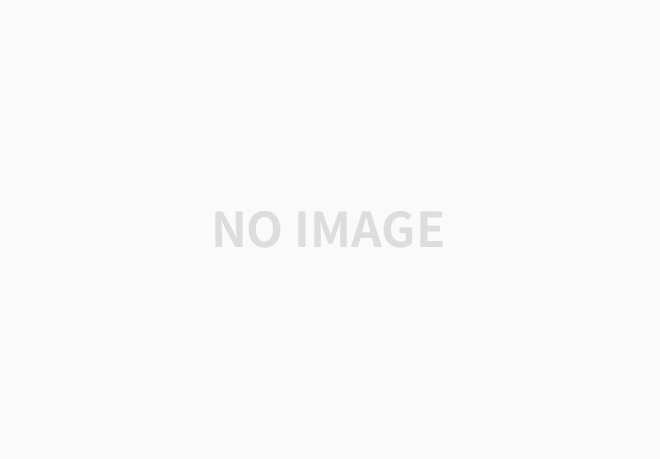
1. multiset
multiset은 중복된 원소를 저장할 수 있다는 점 외에는 set과 동일한 특성을 갖는다.
multiset 또한 기본적으로 오름차순으로 정렬되며 조건자로 greater를 사용함으로써 내림차순으로 정렬할 수 있다.
[STL] Set 생성, 삽입, 삭제 등 사용법
1. set set은 특정 기준에 의하여 원소들이 자동 정렬되는 노드 기반 컨테이너이다. set은 기본적으로 오름차순(less) 정렬이고 greater 조건자를 줌으로써 내림차순으로 정렬할 수도 있다. set은 유
notepad96.tistory.com
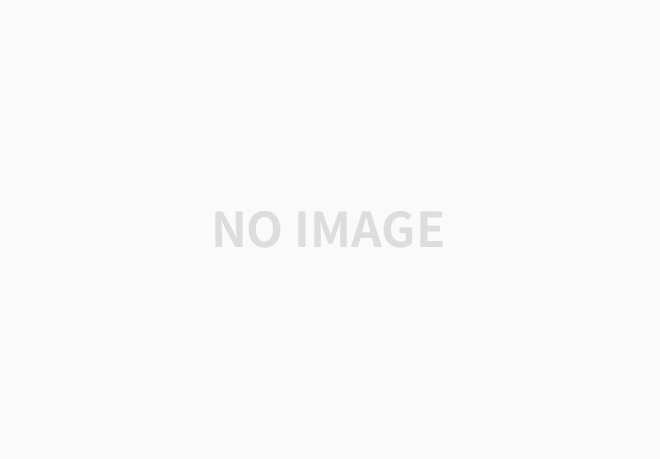
그래서 set이 집합을 의미했다면 multiset은 중복집합을 의미한다는 것을 알 수 있다.
따라서 수학적인 문제를 해결할 시 집합이 필요하다면 set을 중복집합이 필요하다면 multiset을 사용하면 될 것이다.
2. 코 드
#include <iostream>
#include <set>
using namespace std;
int main() {
/* 생성자 */
//multiset<int> mset; // 오름차순, 기본 값 : less<int>
multiset<int, greater<int>> mset; //내림차순
/* 삽입, 삭제 */
mset.insert(30);
mset.insert(50);
mset.insert(50);
mset.insert(40);
mset.insert(10);
mset.insert(20);
mset.insert(40);
mset.insert(50);
mset.erase(20);
for (int i : mset) {
cout << i << " ";
}
cout << "\n";
for (auto it = mset.begin(); it != mset.end(); it++) {
cout << *it << " ";
}
cout << "\n";
/* 값 탐색 */
// 1. 개수 탐색. multiset은 중복허용이므로 1이상의 값도 얻을 수 있다.
cout << "원소 50 개수 : " << mset.count(50) << "\n";
// 2. find 함수로 탐색
if (mset.find(50) == mset.end()) { // find는 존재하지 않으면 mset.end()를 리턴
cout << "원소 50은 현재 없다.\n";
}
else {
cout << "원소 50은 현재 존재한다.\n";
}
// 3. equal_range = pair< lower_bound(), upper_bound() >
auto range = mset.equal_range(40);
cout << *mset.lower_bound(40) << "\n"; // 40의 시작 반복자
cout << *mset.upper_bound(40) << "\n"; // 40의 종료 반복자
for (auto it = range.first; it != range.second; it++) {
cout << *it << " ";
}
cout << "\n";
return 0;
}
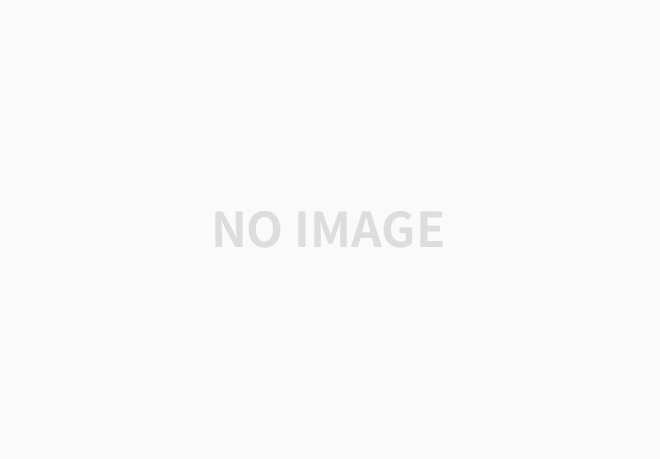
- multiset은 set 라이브러리의 같이 포함되어 있으므로 set을 include한다면 사용할 수 있다.
- count 멤버함수는 set에서 0 또는 1의 값만 가질 수 있었다면 중복이 허용되는 multiset에서는 1이상의 원소의 개수를 얻을 수 있다.
- equal_range() 는 lower_bound() 와 upper_bound()의 쌍(pair) 값을 반환해 준다.
lower_bound() 멤버 함수는 해당 원소의 시작 반복자를 반환하며
upper_bound() 멤버 함수는 해당 원소의 종료 반복자를 반환한다.
이러한 반복자 값을 이용하여서 어느 원소보다 크거나 작은 범위에 있는 원소들을 구할 수도 있다.
여기서 주의할 점은 [ lower_bound(). upper_bound() ) 의 범위를 갖는다는 것이다.
3. 참 조
multiset - C++ Reference
difference_typea signed integral type, identical to: iterator_traits ::difference_type usually the same as ptrdiff_t
www.cplusplus.com
'C++ > Container' 카테고리의 다른 글
[STL] Multimap 생성, 삽입, 삭제 등 사용법 (0) | 2020.11.06 |
---|---|
[STL] Map 생성, 삽입, 삭제 등 사용법 (0) | 2020.11.06 |
[STL] Set 생성, 삽입, 삭제 등 사용법 (0) | 2020.11.05 |
[STL] List 생성, 삽입, 삭제 등 사용법 (0) | 2020.11.04 |
[STL] Deque 생성, 삽입, 삭제 등 사용법 (0) | 2020.11.04 |