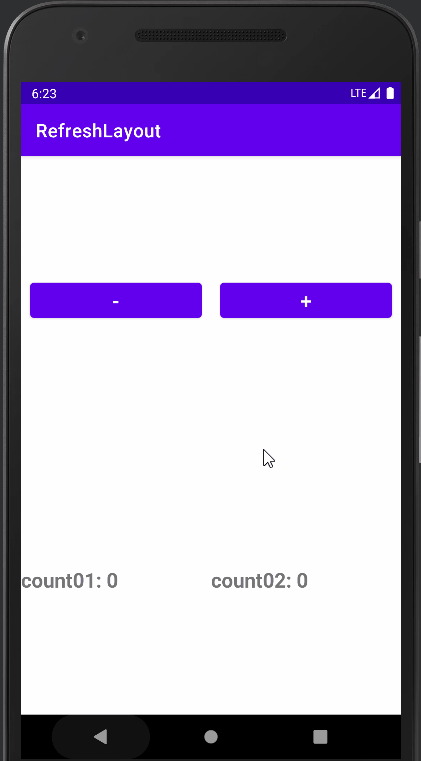
[Android/Kotlin] SwipeRefreshLayout - 내려서 새로고침
Notepad96
·2022. 9. 11. 22:12
1. 요약
이번 글에서는 Layout을 아래로 Swipe 하여 새로고침이 가능한 SwipeRefreshLayout에 관하여 기술한다.
SwipeRefeshLayout을 사용하기 위해서는 아래 종속성을 build.gradle(. app) dependencies의 추가해주어야 한다.
dependencies {
implementation("androidx.swiperefreshlayout:swiperefreshlayout:1.1.0")
}
Swiperefreshlayout | Android 개발자 | Android Developers
알림 이 페이지를 개발자 프로필에 저장하여 중요 업데이트에 대한 알림을 받으세요. 컬렉션을 사용해 정리하기 내 환경설정을 기준으로 콘텐츠를 저장하고 분류하세요. Swiperefreshlayout 스와이
developer.android.com
SwipeRefreshLayout을 사용하면 간편하게 Swipe 하여 Refresh가 가능한 레이아웃을 만들 수 있으며 개발자는 Refresh 할 경우 원하는 동작만을 정의해주면 되기 때문에 유용하게 사용할 수 있다.
2. 레이아웃
2-1. activity_main.xml
메인 레이아웃으로서 위 종속성에서 추가한 SwipeRefreshLayout을 사용하여 레이아웃을 구성하였다.
SwipeRefreshLayout을 사용함으로써 Layout을 아래로 Swipe 함으로써 Refresh를 할 수 있도록 기본적인 기능은 다 구현이 되며, 사용자는 Refresh 함으로써 변경하고자 하는 동작만을 정의해주면 된다.
추가적으로 새로고침이 정상적으로 동작하는지 확인하기 위해서 숫자형 변수 2개의 값을 선언할 것이며, 변경할 수 있도록 Button 2개와 값을 나타내기 위해서 사용할 TextView 2개로 구성하였다.
<?xml version="1.0" encoding="utf-8"?>
<androidx.swiperefreshlayout.widget.SwipeRefreshLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/refreshLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:layout_weight="1">
<Button
android:id="@+id/button01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_marginHorizontal="10dp"
android:text="-"
android:textSize="22sp"/>
<Button
android:id="@+id/button02"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_marginHorizontal="10dp"
android:text="+"
android:textSize="22sp"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:layout_weight="1">
<TextView
android:id="@+id/textView01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="count01: 0"
android:textStyle="bold"
android:textSize="22sp"/>
<TextView
android:id="@+id/textView02"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="count02: 0"
android:textStyle="bold"
android:textSize="22sp"/>
</LinearLayout>
</LinearLayout>
</androidx.swiperefreshlayout.widget.SwipeRefreshLayout>
3. 코드 및 설명
3-1. MainActivity.kt
Button을 클릭할 때마다 숫자형 변수인 count01와 count02의 값을 변화시키도록 하였다.
count01, count02 각 값들은 Textview01, TextView02로 나타내며 Button을 클릭할 때마다 count01은 1씩 증감을 하며 count02는 10씩 증감을 하도록 구현하였다.
새로고침 할 경우 데이터를 새로 불러오거나, 레이아웃을 변경하거나 원하는 동작을 정의할 수 있으며 새로고침 했을 때 동작을 정의하기 위해서는 RefreshLayout의 setOnRefreshListenser를 정의해 준다.
해당 예시에서는 count01을 0으로 초기화하면서 count01, count02 값을 각 TextView의 나타내며 Toast 메시지를 보여주도록 정의하였다.
결과를 확인해보면 count01은 0으로 변경하는 것을 볼 수 있으며 count02는 증감된 만큼 값이 TextView에 나타나는 것을 확인할 수 있다.
처리하고자 하는 작업이 끝났다면 마지막으로 isRefreshing을 false로 변경해주어야 한다. 그렇지 않으면 아래처럼 새로고침 중인 상태가 지속되어 새로고침이 불가해진다.
package com.notepad96.refreshlayout
import android.os.Bundle
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
import com.notepad96.refreshlayout.databinding.ActivityMainBinding
class MainActivity : AppCompatActivity() {
private val binding: ActivityMainBinding by lazy { ActivityMainBinding.inflate(layoutInflater) }
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(binding.root)
var count01 = 0
var count02 = 0
binding.button01.setOnClickListener {
binding.textView01.text = "count01: ${--count01}"
count02 -= 10
}
binding.button02.setOnClickListener {
binding.textView01.text = "count01: ${++count01}"
count02 += 10
}
binding.refreshLayout.setOnRefreshListener {
count01 = 0
binding.textView01.text = "count01: $count01"
binding.textView02.text = "count02: $count02"
Toast.makeText(applicationContext, "Refresh Success", Toast.LENGTH_SHORT).show()
binding.refreshLayout.isRefreshing = false
}
}
}
4. 전체 파일
GitHub - Notepad96/BlogExample02
Contribute to Notepad96/BlogExample02 development by creating an account on GitHub.
github.com
'Android' 카테고리의 다른 글
[Android/Kotlin] AlarmManager + Notification - 지정한 시간에 알림 생성 (0) | 2022.09.15 |
---|---|
[Android/Kotlin] RecyclerView + SwipeRefreshView - 리스트 새로고침 (0) | 2022.09.12 |
[Android/Kotlin] 알림(Notification) 만들기 (1) | 2022.09.10 |
[Android/Kotlin] AlarmManager - 알람 등록 (2) | 2022.09.09 |
[Android/Kotlin] Floating Button Animation (0) | 2022.09.08 |