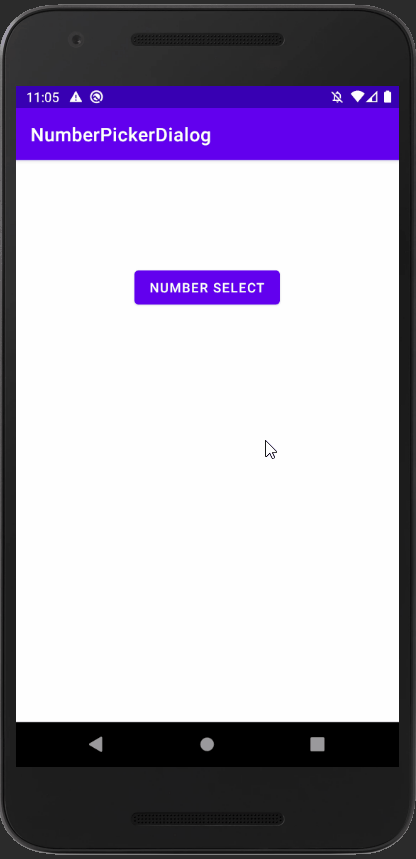
Android Kotlin Number Picker Dialog - 숫자 선택 창
Notepad96
·2022. 2. 7. 09:06
1. 결 과
# 이 글은 숫자를 선택할 수 있도록 하는 Number Picker를 Dialog로 호출하는 방법을 기술한다.
2. activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/btn_number_select"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Number Select"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.203" />
</androidx.constraintlayout.widget.ConstraintLayout>
# 메인 레이아웃은 Dialog를 호출하는 버튼 1개로 구성된다.
2.1 dialog_num_select.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<NumberPicker
android:id="@+id/number_picker"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/btn_cancel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="취 소"
android:textSize="18sp" />
<Button
android:id="@+id/btn_ok"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="확 인"
android:textSize="18sp" />
</LinearLayout>
</LinearLayout>
# Dialog 레이아웃은 숫자를 선택할 수 있게 하는 NumberPicker와 취소/확인의 2개 버튼으로 구성한다.
# Custom Dialog를 생성하는 방법을 응용하여 숫자를 선택할 수 있는 Dialog를 만들어낸다.
Android Kotlin - AlertDialog(알림창) 기본 및 커스텀
1. 결과 2. Custom Dialog Layout (layout/custom_dialog.xml) <?xml version="1.0" encoding="utf-8"?> 3. MainActivity.kt package com.example.alertdialog import android.content.DialogInterface import a..
notepad96.tistory.com
3. MainActivity.kt
package com.example.numberpickerdialog
import android.app.AlertDialog
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
import kotlinx.android.synthetic.main.dialog_num_select.view.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
var selectNum = 0
btn_number_select.setOnClickListener {
val layout = layoutInflater.inflate(R.layout.dialog_num_select, null)
val build = AlertDialog.Builder(it.context).apply {
setView(layout)
}
val dialog = build.create()
dialog.show()
layout.number_picker.minValue = 1
layout.number_picker.maxValue = 100
if(selectNum != 0) layout.number_picker.value = selectNum
layout.btn_cancel.setOnClickListener {
dialog.dismiss()
}
layout.btn_ok.setOnClickListener {
selectNum = layout.number_picker.value
btn_number_select.text = "$selectNum 번"
dialog.dismiss()
}
}
}
}
# numberPicker의 minValue/maxValue를 사용하여 선택 가능한 숫자의 최소값/최대값을 지정할 수 있다.
# numberPicker의 value 값으로 선택한 숫자 값을 읽거나 쓸 수가 있다.
한번 숫자를 선택한 적이 있다면 그 숫자부터 시작하는 것이 자연스럽기 때문에 value 을 설정해주면 된다.
4. 전체 코드
GitHub - Notepad96/BlogExample
Contribute to Notepad96/BlogExample development by creating an account on GitHub.
github.com
'Android' 카테고리의 다른 글
Android Kotlin Image Full Screen - 이미지 클릭 시 확대 (0) | 2022.02.22 |
---|---|
Android Loading Animation - ContentLoadingProgressBar (0) | 2022.02.10 |
Android Kotlin Permission Check - 권한 요청 및 설정 (0) | 2022.02.04 |
Android Kotlin Camera - 사진 찍고 불러오기 (0) | 2022.02.03 |
Android Kotlin Font - 폰트 적용 (0) | 2022.02.02 |