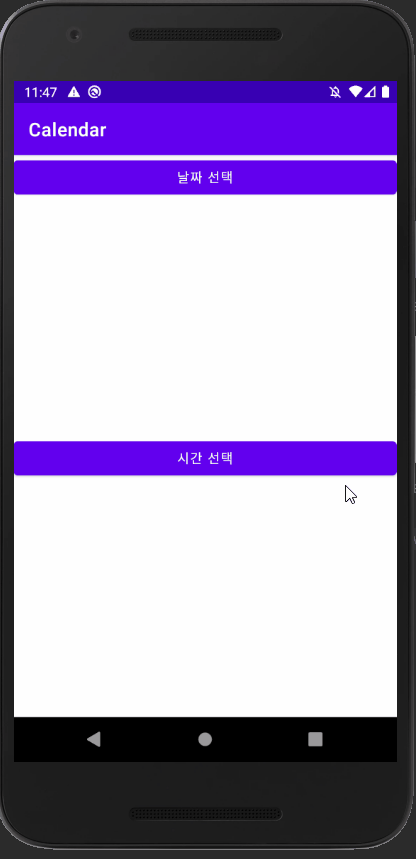
Android Kotlin Calendar - 달력/시계 날짜 및 시간 지정하기
Notepad96
·2022. 1. 18. 23:48
1. 결 과
# 이 글은 DatePickerDialog와 TimePickerDialog를 사용하여 날짜 및 시간을 선택할 수 있는 Dialog를 호출함으로써 선택된 날짜와 시간을 가져올 수 있는 방법을 기술한다.
# 단순히 날짜와 시간을 선택하게하고자 할 때 간단하게 사용할 수 있는 방법이다.
2. activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:id="@+id/btn_load_calendar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="날짜 선택"/>
<TextView
android:id="@+id/text_selected_date"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:textSize="20sp"
android:layout_weight="1"/>
<Button
android:id="@+id/btn_load_timer"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="시간 선택"/>
<TextView
android:id="@+id/text_selected_time"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:textSize="20sp"
android:layout_weight="1"/>
</LinearLayout>
# 레이아웃은 날짜와 시간 Dialog를 호출하는 Button 2개와, 그 결과를 보여줄 TextView 2개로 구성된다.
3. MainActivity.kt
package com.example.calendar
import android.app.DatePickerDialog
import android.app.TimePickerDialog
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
import java.util.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val cal = Calendar.getInstance()
// 달력 로드
btn_load_calendar.setOnClickListener {
DatePickerDialog(this, DatePickerDialog.OnDateSetListener { datePicker, y, m, d ->
text_selected_date.text = "$y/${m+1}/$d"
}, cal.get(Calendar.YEAR), cal.get(Calendar.MONTH), cal.get(Calendar.DAY_OF_MONTH)).show()
}
// 타이머 로드
btn_load_timer.setOnClickListener {
TimePickerDialog(this, TimePickerDialog.OnTimeSetListener { timePicker, h, m ->
text_selected_time.text = "$h:$m"
}, cal.get(Calendar.HOUR), cal.get(Calendar.MINUTE), true ).show()
}
}
}
# DatePickerDialog와 TimePickerDialog는 각각 달력과 시계 Dialog를 생성하며 형태는 비슷하다.
# 2번 째 파라미터로 OnDateSetListener로 달력에서 날짜를 선택했을 시 동작을 선언해주며 '년/월/일'을 'y/m/d'로 읽을 수 있다.
이 때 월을 나타내는 m은 0~11까지의 수를 갖음으로 +1을 해주어야 우리가 사용하는 1~12의 월을 얻을 수 있다.
# 3~5번 째 파라미터로 준 cal.get(Calendar.YEAR)... 는 달력을 호출 시 디폴트로 선택되어 있는 년/월/일을 지정하며 이 예시에서는 현재의 년/월/일을 구하여 디폴트 값으로 주고 있다.
# TimePickerDialog도 비슷한 구조로 호출이 가능하며 h=시간 m=분이다.
마지막 파라미터로 준 true는 24시간 단위로 표시할지의 여부를 지정한다.
4. 전체 코드
GitHub - Notepad96/BlogExample
Contribute to Notepad96/BlogExample development by creating an account on GitHub.
github.com
'Android' 카테고리의 다른 글
Android Kotlin Custom Calendar - 커스텀 달력 (0) | 2022.02.01 |
---|---|
Android Kotlin Chip Button (0) | 2022.01.31 |
Android Kotlin RecyclerView Parent View Style - 부모 뷰 오브젝트 스타일 변경 (0) | 2022.01.17 |
Android Kotlin SnapHelper - 항목 단위로 스크롤 (0) | 2022.01.16 |
Android Kotlin Recyclerview - 리스트 항목 클릭 시 스타일 변경 (0) | 2022.01.13 |