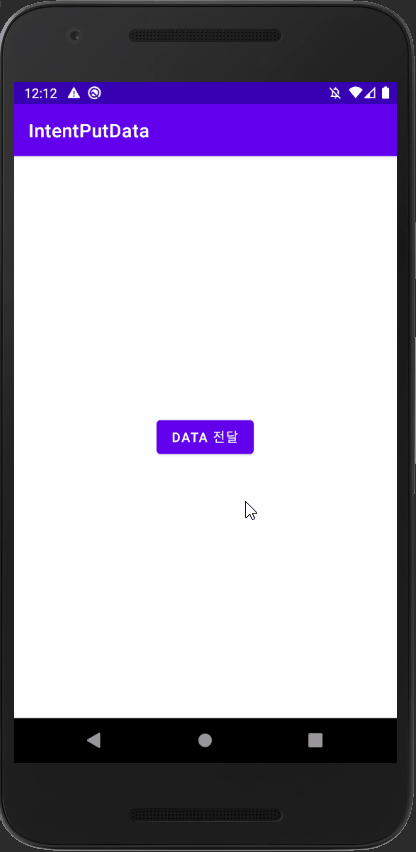
Android Kotlin Intent putExtra, getExtra - 값 전달, 값 받기
Notepad96
·2021. 10. 31. 03:11
300x250
1. 결 과
2. Layout
2-1. activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/btnOpenLayout"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Data 전달"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
2-2. activity_main2.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity2">
<TextView
android:id="@+id/txtGetData"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Test Text"
android:textSize="22sp"
android:textStyle="bold"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
3. MainActivity.kt
package com.example.intentputdata
import android.content.Intent
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
btnOpenLayout.setOnClickListener {
val intent = Intent(applicationContext, MainActivity2::class.java)
intent.putExtra("name", "Lee")
intent.putExtra("age", 32)
startActivity(intent)
}
}
}
# Intent를 생성 한 후 putExtra를 사용하여 넘길 값을 넣는다.
4. MainActivity2.kt
package com.example.intentputdata
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import kotlinx.android.synthetic.main.activity_main2.*
class MainActivity2 : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main2)
val name = intent.getStringExtra("name")
val age = intent.getIntExtra("age", 0)
txtGetData.text = "Name: $name\nAge: $age"
}
}
# intent에 있는 getStringExtra를 사용하여 MainActivity.kt에서 넘긴 String형 name을 받는다.
# intent에 있는 getIntExtra를 사용하여 Int형 값을 받는다. 2번 째 파라미터로는 Default 값을 준다.
5. 전체 코드
GitHub - Notepad96/BlogExample
Contribute to Notepad96/BlogExample development by creating an account on GitHub.
github.com
300x250
'Android' 카테고리의 다른 글
Android Kotlin Snackbar - 안내 메시지 표시 (0) | 2021.11.01 |
---|---|
Android Kotlin Intent Class Data put, get - Class Data 값 전달, 값 받기 (0) | 2021.10.31 |
Android Kotlin Double Click Close - 두 번 클릭하여 종료 (0) | 2021.10.27 |
Android Kotlin RecyclerView Header/Footer - 리사이클러뷰(상하단 레이아웃) (0) | 2021.10.25 |
Android Kotlin RecyclerView Grid - 리사이클러뷰(격자형, 표 형식) (0) | 2021.10.25 |