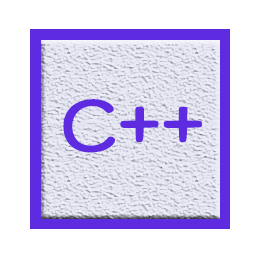
C++ 문자열 소문자, 대문자 변환 transform
Notepad96
·2020. 11. 15. 04:53
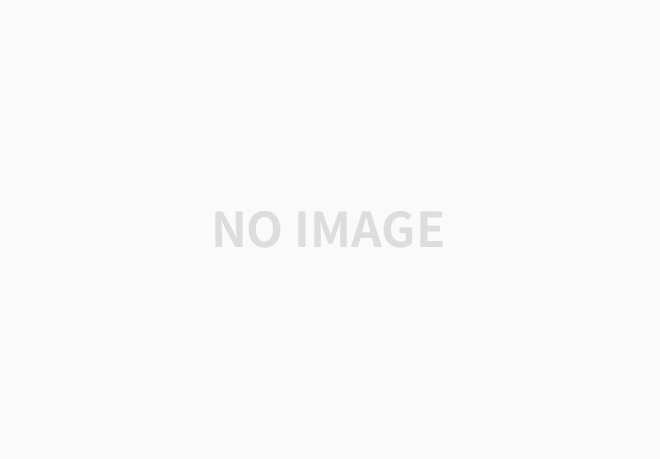
1. transform
transform은 algorithm 라이브러리의 포함되어 있는 함수이다.
transform을 사용하면 일련의 값들을 특정 조건에 따라서 변환 시킬 수 있다.
또한, 이미 구현되어 있는 tolower이나 toupper을 같이 사용하면 간단하게
대문자를 소문자로, 소문자를 대문자로 변환할 수 있다.
2. 코 드
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
typedef pair<int, string> pis;
int main() {
// 1. 숫자 vector
vector<int> v = { 10, 20, 30, 40, 50 };
for (int i : v) cout << i << " ";
cout << "\n";
// 30 이상의 값이라면 10을 곱한다.
transform(v.begin(), v.end(), v.begin(), [](int n) {
if (n >= 30) return n * 10;
else return n;
});
for (int i : v) cout << i << " ";
cout << "\n================================\n";
// 2. 문자열
string s = "abDGSsdFDSafds";
cout << s << "\n";
transform(s.begin(), s.end(), s.begin(), ::tolower); // 소문자 변환
cout << s << "\n";
transform(s.begin(), s.end(), s.begin(), ::toupper); // 대문자 변환
cout << s << "\n================================\n";
vector<string> vs = { "daFDf", "qwFD", "AVD", "SDFtr" };
for (string s : vs) cout << s << " ";
cout << "\n";
// vector 문자열들 소문자 변환
for (int i = 0; i < vs.size(); i++) {
transform(vs[i].begin(), vs[i].end(), vs[i].begin(), ::tolower);
}
for (string s : vs) cout << s << " ";
cout << "\n";
}
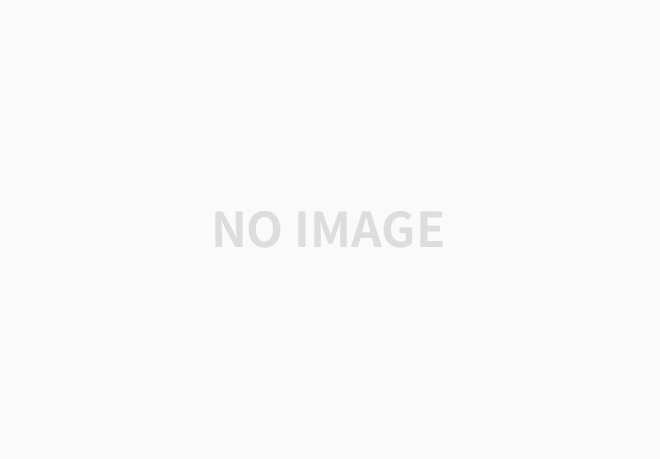
- 1. 숫자 vector의 경우 람다 함수를 사용하여서 30이상의 원소인 경우 10을 곱한 값으로 바꾸도록 하였다.
- 2. 문자열 string 또한 vector처럼 반복자를 제공하므로 이를 사용하여 이미 구현되어 있는
::tolwer 소문자로 변환
::toupper 대문자로 변환
를 사용하면 간단하게 소문자, 대문자로 변환할 수 있다.
3. 참 조
transform - C++ Reference
binary operation(2)template OutputIterator transform (InputIterator1 first1, InputIterator1 last1, InputIterator2 first2, OutputIterator result, BinaryOperation binary_op);
www.cplusplus.com
'C++ > STL' 카테고리의 다른 글
C++ 값 변경하기 replace (0) | 2020.11.16 |
---|---|
C++ 인접하는 중복되는 원소 지우기 unique (0) | 2020.11.15 |
C++ 값 교환하기 Swap (0) | 2020.11.14 |
C++ vector 범위 초기화 Copy (0) | 2020.11.14 |
C++ 동일 원소 포함 여부 확인 is_permutation (0) | 2020.11.14 |