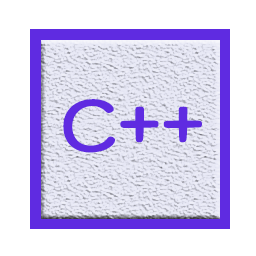
C++ 원소 개수 구하기 Count
Notepad96
·2020. 11. 13. 05:25
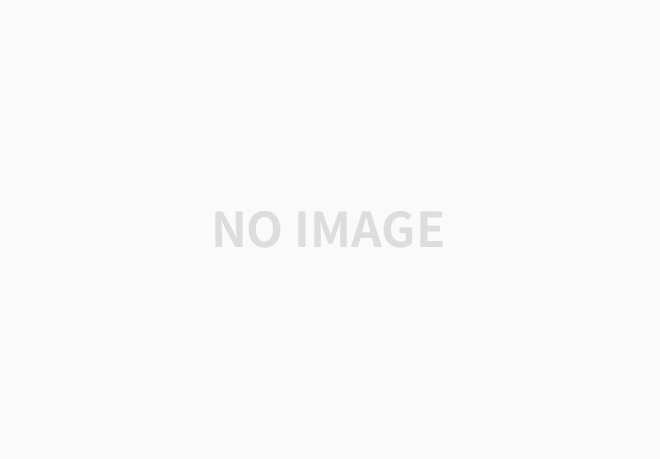
1. count, count_if
algorithm 라이브러리의 count, count_if 함수를 사용한다면
vector나 set와 같은 컨테이너에서 특정 원소가 몇 개 존재하는지 혹은 특정 조건의 만족하는 원소가 몇 개 포함되어 있는지를 구할 수 있다.
사용법은 마찬가지로 algorithm의 포함되어 있는 find, find_if 와 유사하다.
C++ Vector 값 탐색 find - 존재 유무 확인
1. find, find_if vector에서 특정 데이터가 존재하는지 확인하고 싶다. 그렇다면 algorithm 라이브러리의 find를 사용할 수 있다. find는 반복자를 인자로 갖으면서 배열, vector, deque 처럼 일련의 데이터..
notepad96.tistory.com
2. 코 드
환경 : Visual studio 2019
#include <vector>
#include <algorithm>
#include <iostream>
#include <set>
using namespace std;
bool isOdd(int n) {
return n % 2 == 1;
}
int main() {
vector<int> v;
v.push_back(46);
v.push_back(67);
v.push_back(184);
v.push_back(4);
v.push_back(17);
v.push_back(53);
v.push_back(4);
cout << "현재 vector : ";
for (int i : v) cout << i << " ";
cout << "\n==============================\n";
int num = 4;
cout << "원소 "<< num << "의 개수 : " << count(v.begin(), v.end(), num) << "\n";
num = 6;
cout << "원소 " << num << "의 개수 : " << count(v.begin(), v.end(), num) << "\n";
cout << "홀수인 원소의 개수 : " << count_if(v.begin(), v.end(), isOdd) << "\n";
cout << "==============================\n";
set<int> set;
set.insert(4);
set.insert(4);
set.insert(4);
set.insert(7);
set.insert(5);
num = 4;
cout << "원소 " << num << "의 개수 : " << count(set.begin(), set.end(), num) << "\n";
cout << "홀수인 원소의 개수 : " << count_if(set.begin(), set.end(), isOdd) << "\n";
return 0;
}
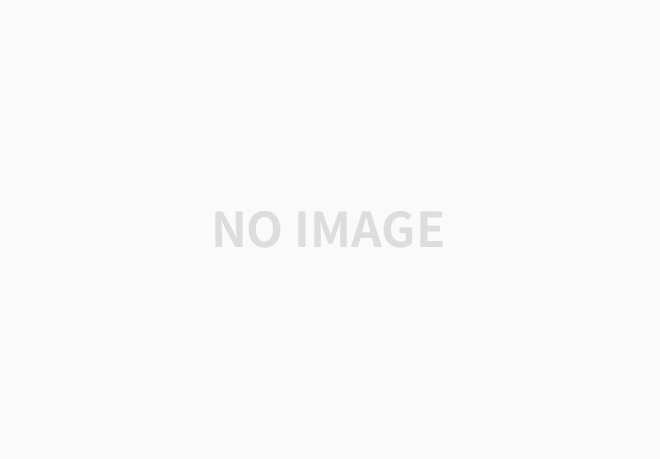
- count 함수는 인자로 탐색할 범위의 시작과 끝 반복자와 탐색할 원소를 받는다.
해당 원소가 존재하지 않을 경우 0을 반환한다.
- set의 경우 중복된 원소가 삽입되지 않으므로 count로 원소 개수를 구하여도 0또는 1만을 얻는다.
- count가 탐색할 원소를 받았다면 count_if는 조건을 인수로 받아 조건의 만족하는 원소의 개수를 반환한다.
3. 참 조
count - C++ Reference
function template std::count template typename iterator_traits ::difference_type count (InputIterator first, InputIterator last, const T& val); Count appearances of value in range Returns the number of elements in the range [first,last) that co
www.cplusplus.com
count_if - C++ Reference
function template std::count_if template typename iterator_traits ::difference_type count_if (InputIterator first, InputIterator last, UnaryPredicate pred); Return number of elements in range satisfying condition Returns the number of elements
www.cplusplus.com
'C++ > STL' 카테고리의 다른 글
C++ 동일 원소 포함 여부 확인 is_permutation (0) | 2020.11.14 |
---|---|
C++ 일련하는 원소 찾기 Search (0) | 2020.11.13 |
C++ Vector 값 탐색 find - 존재 유무 확인 (0) | 2020.11.12 |
C++ Vector 최대값, 최소값, 인덱스 구하기 (0) | 2020.11.12 |
C++ 조합(Combination) - next_permutation (1) | 2020.11.12 |