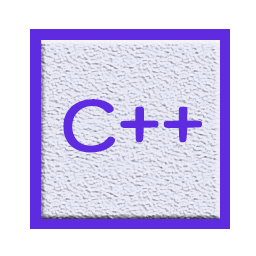
C++ 조합(Combination) - next_permutation
Notepad96
·2020. 11. 12. 05:45
1. 조합(Combination)
조합(Combination)이란 n개의 원소를 갖는 집합에서 m(n 이하의 자연수)개를 선택하여 만드는 부분집합들이다.
C++에서는 algorithm 라이브러리의 next_permutation을 사용하면 이를 쉽게 구할 수 있다.
본래 next_permutation은 순열(Permutation)을 구할 때 사용하였었다.
C++ 순열(Permutation) - next_permutation
1. 순열(Permutation) 순열은 순서에 상관있게 값들을 나열하는 것을 의미한다. C++ 에서는 algorithm 라이브러리의 next_permutation을 사용한다면 간단하게 구해낼 수 있다. next_permutation은 인자로 반복..
notepad96.tistory.com
이런 순열(Permutation)의 특성을 이용하면 조합(Combination) 또한 구할 수 있다.
2. 코 드
환경 : Visual studio 2019
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
using namespace std;
int main() {
string s = "12345";
int len = s.size();
for (int i = 1; i <= len; i++) {
vector<bool> v(len - i, false);
v.insert(v.end(), i, true);
cout << "=============== " << i << "개 ===============\n";
do {
string temp = "";
for (int k = 0; k < len; k++) {
if (v[k]) temp += s[k];
}
cout << temp << "\n";
} while (next_permutation(v.begin(), v.end()));
cout << "===================================\n";
}
return 0;
}

- 해당 예시에서는 간단하게 문자열을 사용하였다. 이를 vector로 바꾸거나한다면 집합에서 조합을 구하는 것처럼 할 수 있다.
- vector v는 집합(여기서는 s)의 크기만큼을 갖으며, i는 선택할 원소의 개수이다.
따라서 s의 길이는 5이므로 1~5까지 for루프를 돌면서 원소를 1개 선택하는 경우, 2개 선택하는 경우, ... ,5개 선택하는 겨우를 각각 구할 수 있는 것이다.
i : v
1 : false, false, false, false, true => 5개 중 1개 선택하는 경우
2 : false, false, false, true, true => 5개 중 2개 선택하는 경우
3 : false, false,true, true, true=> 5개 중 3개 선택하는 경우
4 : false, true, true, true, true => 5개 중 4개 선택하는 경우
5 : true, true, true, true, true => 5개 중 5개 선택하는 경우
- do~while 문을 이용하여 next_permutation을 사용한다.
next_permutation에 의하여 v의 순서가 바뀌며 v의 원소 중 true일 경우 같은 인덱스의 위치하는 문자열 s의 문자를 합치면 조합을 구해낼 수 있다.
3. 참 조
[STL] Vector 생성, 삽입, 삭제 등 사용법
1. vector vector은 배열 기반 컨테이너이다. 따라서 배열과 비슷하여 사용하기 쉬워서 빈번하게 사용된다. 하나의 메모리 블록에 연속하여 저장되는 특징을 갖는다. 연속하여 저장되어 원소에 접근
notepad96.tistory.com
next_permutation - C++ Reference
function template std::next_permutation default (1)template bool next_permutation (BidirectionalIterator first, BidirectionalIterator last); custom (2)template bool next_permutation (BidirectionalIterator first, BidirectionalIterator last, Comp
www.cplusplus.com
'C++ > STL' 카테고리의 다른 글
C++ 원소 개수 구하기 Count (0) | 2020.11.13 |
---|---|
C++ Vector 값 탐색 find - 존재 유무 확인 (0) | 2020.11.12 |
C++ Vector 최대값, 최소값, 인덱스 구하기 (0) | 2020.11.12 |
C++ 순열(Permutation) - next_permutation (0) | 2020.11.11 |
C++ vector 정렬(sort) - 오름차순, 내림차순 (0) | 2020.11.10 |