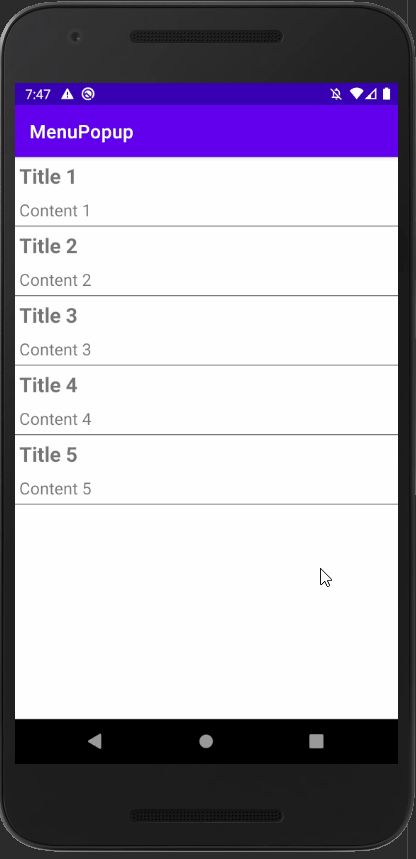
Android Kotlin Pop Menu - 메뉴 생성
Notepad96
·2021. 12. 26. 20:16
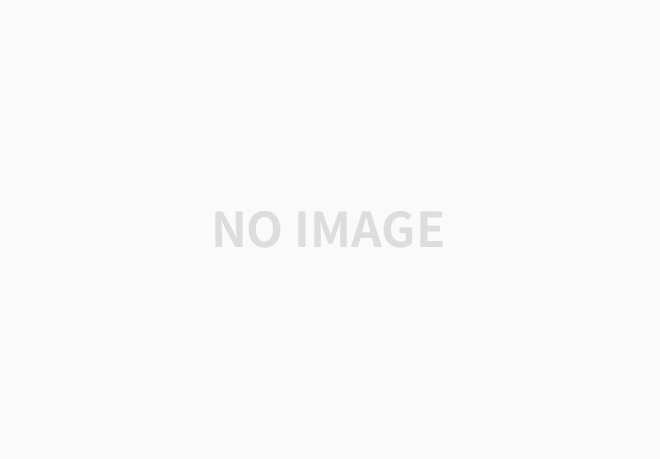
1. 결 과
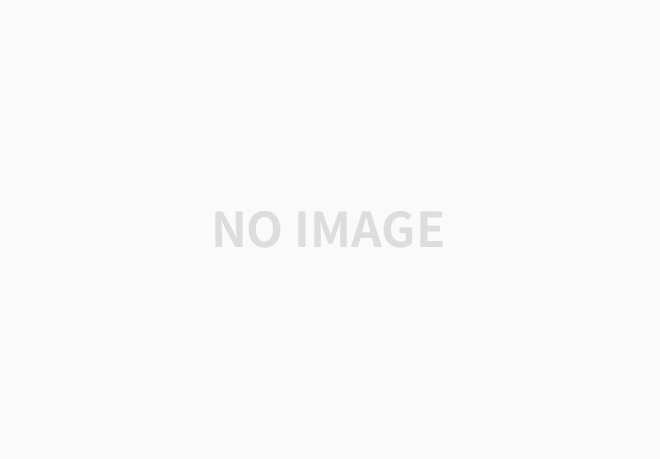
# 해당 글은 Popup menu를 생성하고 항목을 Click하였을 시 이벤트 처리하는 방법을 기술한다.
# popup menu는 특정 콘텐츠와 관련된 작업에 더 보기 스타일 메뉴를 제공하는 경우 유용하게 사용이 가능하다.
# context menu와 다르게 click 시 혹은 long click 시 동작하도록 할 수 있다.
2. activity_main.xml (메인 Layout)
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/vListMain"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
# 메인 레이아웃은 리스트를 보여줄 RecyclerView 하나로 구성된다.
3. list_item_popup.xml (popup 메뉴)
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:title="속 성" android:id="@+id/mItem01"/>
<item android:title="수 정" android:id="@+id/mItem02" />
<item android:title="삭 제" android:id="@+id/mItem03" />
</menu>
# popup menu로 보여줄 menu로서 3개의 항목으로 구성된다.
# menu 파일은 res아래 menu directory을 만든 후 그 안에 menu 파일을 생성한다.
4. List
4-1. mylist_item.xml (List Item Layout)
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/vLayoutMyListItem"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/vTextMyListTitle"
android:padding="5dp"
android:text="Test Title"
android:textSize="22sp"
android:textStyle="bold" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/vTextMyListContent"
android:padding="5dp"
android:text="Test Contents"
android:textSize="18sp" />
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="#777"/>
</LinearLayout>
# List의 보여줄 항목(Item)의 레이아웃이며, TextView 2개로 구성된다.
4-2. MyListAdapter.kt (List Adapter)
package com.example.menupopup
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.PopupMenu
import android.widget.Toast
import androidx.recyclerview.widget.RecyclerView
import kotlinx.android.synthetic.main.mylist_item.view.*
class MyListAdapter: RecyclerView.Adapter<MyListAdapter.MyView>() {
private val testTitleList = listOf("Title 1", "Title 2", "Title 3", "Title 4", "Title 5" )
private val testContentList = listOf("Content 1", "Content 2", "Content 3", "Content 4", "Content 5")
inner class MyView(val layout: View): RecyclerView.ViewHolder(layout)
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): MyView {
return MyView(LayoutInflater.from(parent.context).inflate(R.layout.mylist_item, parent, false))
}
override fun onBindViewHolder(holder: MyView, position: Int) {
holder.layout.vTextMyListTitle.text = testTitleList[position]
holder.layout.vTextMyListContent.text = testContentList[position]
holder.layout.vLayoutMyListItem.setOnClickListener {
val popup = PopupMenu(holder.layout.context, it)
popup.menuInflater.inflate(R.menu.list_item_popup, popup.menu)
popup.setOnMenuItemClickListener { item ->
var text = when(item.itemId) {
R.id.mItem01 -> "${position+1}번 째 속성 Click"
R.id.mItem02 -> "${position+1}번 째 수정 Click"
else -> "${position+1}번 째 삭제 Click"
}
Toast.makeText(holder.layout.context, text, Toast.LENGTH_LONG).show()
true
}
popup.show()
}
}
override fun getItemCount(): Int {
return testTitleList.size
}
}
# List의 Adapter를 재정의 한다.
# item의 Layout을 클릭하였을 시 popup 메뉴를 생성하도록 구성하였다.
# popup의 setOnMenuItemClickListener을 사용하면 메뉴의 항목 클릭 시 이벤트를 정의할 수 있다.
5. MainActivity.kt
package com.example.menupopup
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.recyclerview.widget.LinearLayoutManager
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
var listManager = LinearLayoutManager(this)
var listAdapter = MyListAdapter()
vListMain.apply {
layoutManager = listManager
adapter = listAdapter
}
}
}
# 메인 레이아웃의 구성하였던 RecyclerView의 adapter와 layoutmanager를 선언한 후 연결하여 리스트를 구성한다.
6. 전체 코드 & 참 조
GitHub - Notepad96/BlogExample
Contribute to Notepad96/BlogExample development by creating an account on GitHub.
github.com
메뉴 | Android 개발자 | Android Developers
메뉴 메뉴는 수많은 유형의 애플리케이션에서 사용되는 보편적인 사용자 인터페이스 구성요소입니다. 친숙하고 일관적인 사용자 경험을 제공하기 위해 Menu API를 사용하여 활동에서 사용자 작
developer.android.com
'Android' 카테고리의 다른 글
Android Kotlin Recyclerview - 리스트 항목 클릭 시 스타일 변경 (0) | 2022.01.13 |
---|---|
Android Kotlin Bottom Navigation - 하단 네비게이션 (0) | 2022.01.12 |
Android Kotlin Context Menu - 컨텍스트 메뉴 (0) | 2021.12.22 |
Android Kotlin Option Menu - 옵션 메뉴 (0) | 2021.12.21 |
Android Kotlin Splash Page - 시작 페이지, 로고 페이지 (0) | 2021.12.20 |