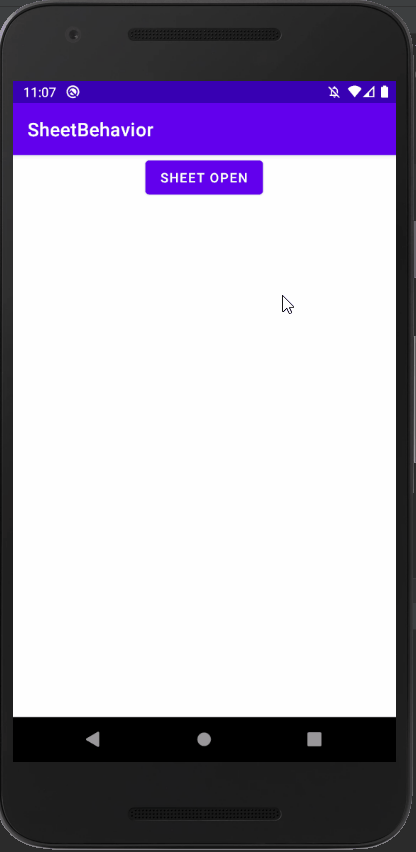
Android Kotlin BottomSheetBehavior - 하단 시트 띄우기
Notepad96
·2021. 10. 10. 23:51
1. 결 과
2. activity_main.xml (메인 레이아웃)
<?xml version="1.0" encoding="utf-8"?>
<androidx.coordinatorlayout.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/layoutMain"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center">
<Button
android:id="@+id/btnOpenSheet"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Sheet Open" />
</LinearLayout>
<LinearLayout
android:id="@+id/bottomSheet"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:maxHeight="100dp"
app:layout_behavior="@string/bottom_sheet_behavior"
app:behavior_peekHeight="80dp"
app:behavior_hideable="true">
<include
android:id="@+id/layoutDialog"
layout="@layout/sheet_main"/>
</LinearLayout>
</androidx.coordinatorlayout.widget.CoordinatorLayout>
# BottomSheetBehavior을 사용하기 위해서는 보여주고자 하는 레이아웃이 CoordinatorLayout 안에 포함되어 있어야 한다.
# 동작은 Button을 클릭 시 하단 시트가 보이도록 하였다.
# app:layout_behavior="@string/bottom_sheet_behavior" : behavior을 지정
# app:behavior_peekHeight : 레이아웃 높이를 지정한다. 시트의 길이보다 낮게 지정할 일부의 레이아웃만 보여주며 이를 위로 스크롤 하였을 때 전체를 보여주도록 할 수 있다.
# app:behavior_hideable : 시트 숨김 가능 여부
3. sheet_main.xml (하단 시트 레이아웃)
<?xml version="1.0" encoding="utf-8"?>
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center"
android:background="#efefef"
android:padding="10dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:text="Width: "/>
<SeekBar
android:id="@+id/seekWidth"
android:layout_width="wrap_content"
android:layout_weight="1"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/textSeekProgress"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="0"
android:textSize="18sp"
android:textStyle="bold"/>
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_marginVertical="8dp"
android:background="#dddddd"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:text="Height: "/>
<SeekBar
android:id="@+id/seekHeight"
android:layout_width="wrap_content"
android:layout_weight="1"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/textSeekProgress2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="0"
android:textSize="18sp"
android:textStyle="bold"/>
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_marginVertical="8dp"
android:background="#dddddd"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:src="@drawable/ic_launcher_foreground"
android:background="@drawable/ic_launcher_background"/>
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Test 01"
android:textSize="22sp"
android:gravity="center"/>
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_marginVertical="8dp"
android:background="#dddddd"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:src="@drawable/ic_launcher_foreground"
android:background="@drawable/ic_launcher_background"/>
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Test 02"
android:textSize="22sp"
android:gravity="center"/>
</LinearLayout>
</LinearLayout>
</ScrollView>
# 가독성을 높이기 위하여 하단 시트를 다른 레이아웃 파일로 구성하였다. 이는 activity_main에서 include를 사용하여 연결해준다.
4. MainActivity.kt
package com.example.sheetbehavior
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import com.google.android.material.bottomsheet.BottomSheetBehavior
import kotlinx.android.synthetic.main.activity_main.*
import kotlinx.android.synthetic.main.sheet_main.view.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
var behavior = BottomSheetBehavior.from(bottomSheet)
layoutDialog.seekWidth.progress = 50
layoutDialog.textSeekProgress.text = "50"
behavior.state = BottomSheetBehavior.STATE_HIDDEN
btnOpenSheet.setOnClickListener {
behavior.state = BottomSheetBehavior.STATE_COLLAPSED
}
layoutMain.setOnClickListener {
behavior.state = BottomSheetBehavior.STATE_HIDDEN
}
}
// STATE_COLLAPSED : peekHeight 만큼
// STATE_EXPANDED : 가득
// STATE_HIDDEN : 숨김
}
# BottomSheetBehavior.from의 파라미터로 시트가 포함된 View를 전달한다.
# behavior.state의 값을 바꾸어 시트를 보이거나 숨기는 것이 가능하다.
- STATE_COLLAPSED : 레이아웃에 지정하였던 peekHeight 만큼 시트를 보인다.
- STATE_EXPANDED : 시트 전체를 보인다.
- STATE_HIDDEN : 시트 전체를 숨긴다.
각 값들은 다음과 같은 동작을 한다.
5. 전체 코드
GitHub - Notepad96/BlogExample
Contribute to Notepad96/BlogExample development by creating an account on GitHub.
github.com
'Android' 카테고리의 다른 글
Android Kotlin RecyclerView Grid - 리사이클러뷰(격자형, 표 형식) (0) | 2021.10.25 |
---|---|
Android Kotlin RecyclerView - 리사이클러뷰(가로, 세로) (1) | 2021.10.24 |
Android Kotlin BottomSheetDialog - 아래 팝업 레이아웃 (0) | 2021.10.09 |
Android Kotlin Click Event (0) | 2021.10.07 |
Android Kotlin id로 View 접근 (0) | 2021.10.07 |