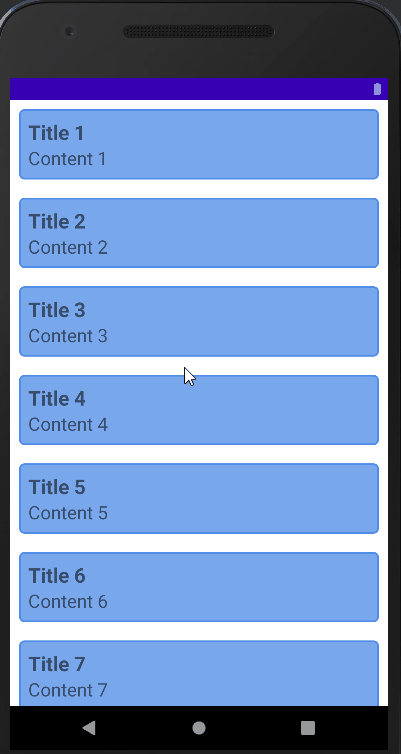
[Android/Kotlin] Recyclerview List Animation(리스트 애니메이션)
Notepad96
·2022. 8. 16. 11:34
1. 요약
이 글에서는 Recyclerview 리스트의 Item들이 Load 될 때 Animation을 설정하는 방법에 관하여 기술한다.
이를 위해서 아래 2가지 내용을 정의할 필요가 있다.
- RecyclerView 리스트를 나타내기 위한 Adapter 정의
- Item의 적용할 Animation 정의 및 적용
Animation 경우 drawable 아래 anim이라는 Resource Directory를 생성한 후 그 안에 Animation 파일을 생성한 후 정의하며 List Adapter서 Layout의 animation 값을 지정함으로 List Item Animation을 구현할 것이다.
2. 레이아웃
2-1. activity_main.xml
메인 레이아웃으로서 1개의 Recyclerview로 구성된다.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recycler"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</LinearLayout>
2-2. list_item.xml
Recyclerview List에 나타낼 Item의 레이아웃이다.
Background를 보면 정의된 스타일을 지정하였으며 이와 관련된 내용은 아래 글을 참고하면 된다.
[TIP] Android Style Background 꾸미기 - 테두리, 모서리 둥글게 등
Android Background 꾸미기 - 테두리(border) 굵기 지정 및 색 변경, 모서리 (corner) 둥글게 지정 /res/drawable 서 리소스 파일 생성 후 작성 <?xml version="1.0" encoding="utf-8"?> # shape의 shape 속성으..
notepad96.tistory.com
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:padding="10dp"
android:background="@drawable/bg_list_item"
android:orientation="vertical">
<TextView
android:id="@+id/text_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Test 1"
android:textSize="22sp"
android:textStyle="bold"/>
<TextView
android:id="@+id/text_content"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Content 1"
android:textSize="20sp"
/>
</LinearLayout>
3. 코드 및 설명
3-1. MyListAdapter.kt
List Adapter 파일로서 전반적인 내용은 기존에 Recyclerview를 정의하는 경우와 동일하여 이와 관련된 설명은 아래 글을 참고하면 될 것 같다.
Android Kotlin RecyclerView - 리사이클러뷰(가로, 세로)
1. 결과 2. activity_main.xml (메인 레이아웃) <?xml version="1.0" encoding="utf-8"?> # 1번 째 리사이클러 뷰는 Vertical(세로) 방향의 리사이클러 뷰 # 2번 째 리사이클러 뷰는 Horizontal(가로) 방향의 리..
notepad96.tistory.com
기존 부분과 다른 부분은 Animation을 적용하기 위해 layout.animation의 AnimationUtils.loadAnimation을 통하여 정의한 Animation 파일을 불러와 초기화해준다.
package com.example.recyclerviewitemanimation
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.view.animation.AnimationUtils
import androidx.recyclerview.widget.RecyclerView
import kotlinx.android.synthetic.main.list_item.view.*
class MyListAdapter: RecyclerView.Adapter<MyListAdapter.MyViewHolder>() {
inner class MyViewHolder(val layout: View): RecyclerView.ViewHolder(layout)
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): MyViewHolder {
val view = LayoutInflater.from(parent.context).inflate(R.layout.list_item, parent, false)
return MyViewHolder(view)
}
override fun onBindViewHolder(holder: MyViewHolder, position: Int) {
holder.layout.animation = AnimationUtils.loadAnimation(holder.layout.context, R.anim.list_item_animation)
holder.layout.text_title.text = "Title ${position+1}"
holder.layout.text_content.text = "Content ${position+1}"
}
override fun getItemCount(): Int {
return 1000
}
}
3-2. list_item_animation.xml
Animation을 정의하는 파일로서 drawable/anim 디렉터리의 파일을 생성한다. anim 디렉터리가 없을 경우 Android Resource Directory를 통하여 생성해 주면 된다.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/decelerate_interpolator">
<!-- <translate android:fromXDelta="-200%"-->
<!-- android:toXDelta="0%"-->
<!-- android:duration="1000"/>-->
<!-- <scale-->
<!-- android:pivotX="50%"-->
<!-- android:pivotY="50%"-->
<!-- android:fromXScale="0"-->
<!-- android:fromYScale="0"-->
<!-- android:toXScale="1"-->
<!-- android:toYScale="1"-->
<!-- android:duration="500" />-->
<alpha
android:fromAlpha="0.0"
android:toAlpha="3.0"
android:duration="2000"/>
</set>
● translate
이동시키는 Animation을 보여줄 수 있으며 fromX = -200%에서 toX = 0%로 함으로써 왼쪽에서 오른쪽으로 Item이 옮겨오듯이 나타나도록 구현을 하였다.
duration은 지속 시간으로서 "1000=1초"이다.
● scale
크기를 조절하는 Animation으로서 fromScale = 0에서 toScale = 1로 지정하여 점점 커지도록 구현하였다.
duration은 위와 마찬가지로 나타나는 시간 값을 나타내며 500으로 지정하여 0.5초에 짧은 시간에 해당 Animation 동작을 하도록 만든다.
● alpha
투명도를 조절하는 기능으로서 투명한 상태에서 점점 나타나도록 Animation을 구현할 수 있다.
4. 전체 파일
GitHub - Notepad96/BlogExample
Contribute to Notepad96/BlogExample development by creating an account on GitHub.
github.com
'Android' 카테고리의 다른 글
[Android/Kotlin] TabLayout + ViewPager2 - Slide하여 화면 전환 (0) | 2022.08.18 |
---|---|
[Android/Kotlin] ViewBinding 뷰 오브젝트 접근 (findViewById 대체) (0) | 2022.08.17 |
[Android/Kotlin] 카메라로 찍은 후 Image 불러오기 (Image 파일 저장) (0) | 2022.08.15 |
[Android][Kotlin] 이미지 불러오기 - registerForActivityResult, coil (0) | 2022.04.30 |
Android Kotlin coil - 이미지 로딩 라이브러리 (0) | 2022.04.28 |