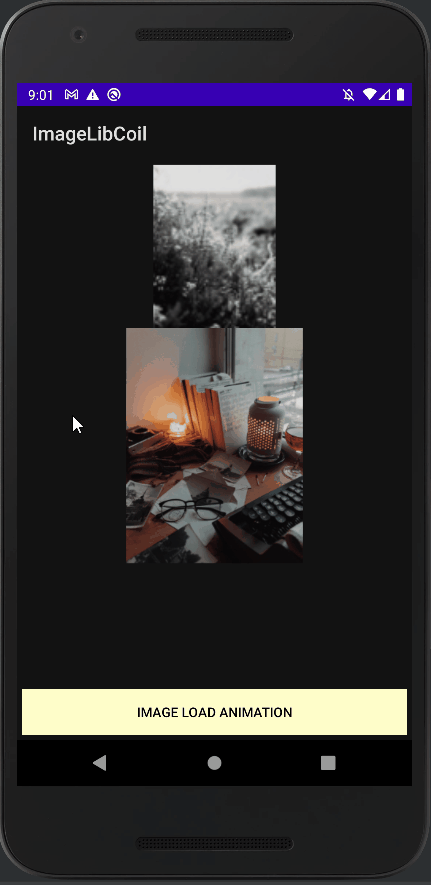
Android Kotlin coil - 이미지 로딩 라이브러리
Notepad96
·2022. 4. 28. 09:41
1. 결 과
# 이 글은 Android서 사용 가능한 이미지 로딩 라이브러리 중 하나인 Coil에 대하여 기술한다.
Coil은 Kotlin Coroutines으로 만들어진 Android 백앤드 이미지 로딩 라이브러리입니다. Coil 은:
- 빠르다: Coil은 메모리와 디스크의 캐싱, 메모리의 이미지 다운 샘플링, Bitmap 재사용, 일시정지/취소의 자동화 등등 수 많은 최적화 작업을 수행합니다.
- 가볍다: Coil은 최대 2000개의 method들을 APK에 추가합니다(이미 OkHttp와 Coroutines을 사용중인 앱에 한하여), 이는 Picasso 비슷한 수준이며 Glide와 Fresco보다는 적습니다.
- 사용하기 쉽다: Coil API는 심플함과 최소한의 boilerplate를 위하여 Kotlin의 기능을 활용합니다.
- 현대적이다: Coil은 Kotlin 우선이며 Coroutines, OkHttp, Okio, AndroidX Lifecycles등의 최신 라이브러리를 사용합니다.
Coil은: Coroutine Image Loader의 약자입니다.
(출처: https://coil-kt.github.io/coil/README-ko/)
Coil 라이브러리를 사용하기 위해서는 build.gradle(app) 파일에
implementation("io.coil-kt:coil:2.0.0-rc03")
를 추가해주어야 한다.
2. activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="5dp"
tools:context=".MainActivity">
<ImageView
android:id="@+id/img_test01"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"/>
<ImageView
android:id="@+id/img_test02"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"/>
<ImageView
android:id="@+id/img_test03"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"/>
<androidx.appcompat.widget.AppCompatButton
android:id="@+id/btn_test"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Image Load Animation"
android:background="#FFFDC9"
/>
</LinearLayout>
# 레이아웃은 이미지를 표시할 이미지뷰 3개와 이미지 애니메이션을 보여줄 버튼 1개로 구성한다.
3. MainActivity.kt
package com.example.imagelibcoil
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import coil.load
import coil.transform.CircleCropTransformation
import coil.transform.GrayscaleTransformation
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
img_test01.load(R.drawable.img01) {
// 1. Circle(둥글게) 처리 CircleCropTransformation()
// transformations(CircleCropTransformation())
// 2. Blur 처리 BlurTransformation(context, radius, sample)
// radius: Blur 반경, sample: 이미지 크기를 조정. 기본값 1로 보다 큰값은 축소하며 작은값은 확대함
// transformations(BlurTransformation(applicationContext, 1f, 1f))
// 3. 회색 처리 GrayscaleTransformation()
transformations(GrayscaleTransformation())
// 4. 모서리 라운드 처리 RoundedCornersTransformation(topLeft, topRight, bottomLeft, bottomRight)
// 모서리 4개의 각각의 코너 값 지정 가능
// transformations(RoundedCornersTransformation(5f, 10f, 15f, 20f))
}
img_test02.load(R.drawable.img02) {
placeholder(R.drawable.img03) // 대체 이미지
error(R.drawable.img03) // Load Error 시 보여줄 이미지
size(300)
}
btn_test.setOnClickListener {
img_test03.load(R.drawable.img03) {
transformations(CircleCropTransformation())
crossfade(2000) // 이미지 fade in Animation
}
}
}
}
# 이미지뷰의 load를 사용함으로써 간단하게 이미지를 보여줄 수 있다.
# 이미지를 보여줄 뿐만 아니라 transformations을 사용하여서
1. CircleCropTransformation: Circle(원형)으로 이미지 표시
2. BlurTransformation: Blur 처리
3. GrayscaleTransformation: 회색 처리
4. RoundedCornersTransformation: 모서리 라운드 처리
같이 특수처리를 할 수 있다.
# 이외에도 placeholder(=대체 이미지 설정), error(=Image Load Error 시 보여줄 이미지), size, crossfade(fade in 애니메이션) 등 다양한 기능을 제공한다.
4. 전체 코드
GitHub - Notepad96/BlogExample
Contribute to Notepad96/BlogExample development by creating an account on GitHub.
github.com
'Android' 카테고리의 다른 글
[Android/Kotlin] 카메라로 찍은 후 Image 불러오기 (Image 파일 저장) (0) | 2022.08.15 |
---|---|
[Android][Kotlin] 이미지 불러오기 - registerForActivityResult, coil (0) | 2022.04.30 |
Android Kotlin Permission - registerForActivityResult (0) | 2022.04.26 |
Android Kotlin Image Full Screen - 이미지 클릭 시 확대 (0) | 2022.02.22 |
Android Loading Animation - ContentLoadingProgressBar (0) | 2022.02.10 |